Fearless Founder Gavin recently finished a Herculean task: setting up SAP Fiori on our AWS development landscape. I would compare this feat to casting the One Ring into the fires of Mount Doom, but Gavin’s really not all that hobbit-like, and he only encountered metaphorical orcs on his journey.
I think I’ve met my mythology/fantasy reference quota for today.
SAP Fiori applications are pretty useful and user-friendly right out of the box. Side-by-side with this initial usefulness is a set of tools and process that you can use to enhance and extend the delivered services with your own business logic. As a developer, the first thing I wanted to do was mess around with stuff anyway – so it’s nice to know that SAP built in ways for me to do that. 🙂
I picked apart one of the apps we have and present below how I went about changing the services that power it.
Things you should note:
- In this example, I’m using the Track Purchase Order application. I’m tackling the service enhancements first, and will post later about the Fiori UI enhancement process.
- SAP publishes an overview of the apps. Note that most of these ERP apps have separate pages detailing their extensibility and identify the specific enhancement points.
- This app is part of the first wave of Fiori apps. I can’t make any promises that this process will fit all next-gen apps, and in fact future releases of Fiori will probably follow a bit more standardized process for extensions – which I’ll make sure to blog about when we get our hands on the next wave.
- I am following some of the things on this SAP page, but I have added some steps of my own as I discovered how to get this done.
- To do the play-at-home version you need the appropriate Fiori installation set up in your ECC system.
- After every step in enhancing things, I made sure to clean up cache on both my ECC and my Gateway systems. I found that sometimes I didn’t see the changes I wanted until I ran /IWBEP/CACHE_CLEANUP on both systems. I recommend this as a general step in creating
Scenario
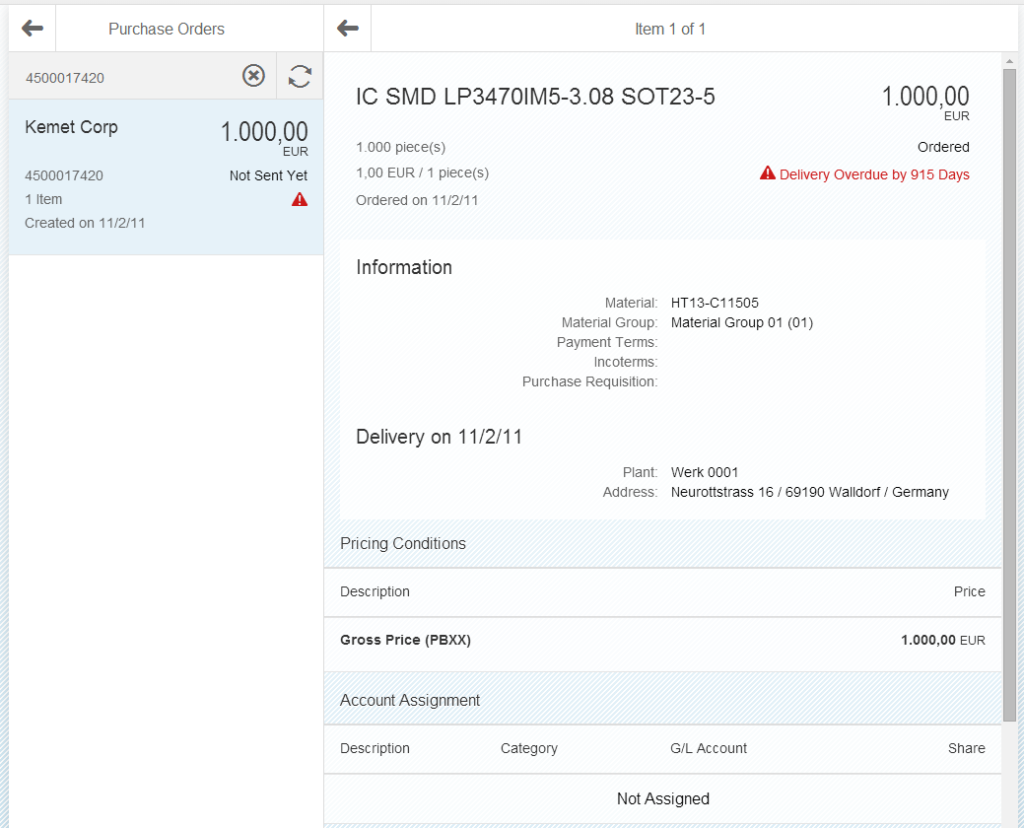
Enhancing the entity
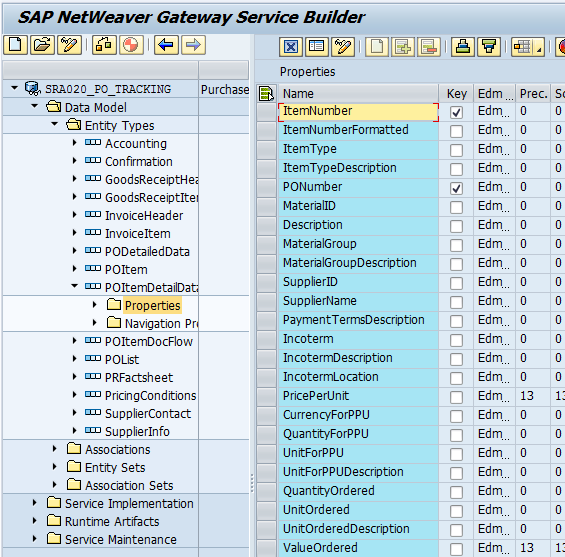
The ABAP dictionary structure that defines this entity is SRA020_S_PO_ITEM_DETAILED. If you open that structure and scroll to the bottom of the components list, you’ll see that SAP has provided an append SRA020_S_PO_ITEM_DETAILED_INCL. For my purposes, I opened this include and created a customer append that held the field I wanted (vtweg, the distribution channel).

Next, you’ll need to create an implementation of BAdI SRA020_PO_TRACKING_MPC. This provides the code hooks to update the runtime definition of the structures of the service. Go to t-code SE18 and enter the BAdI name in the “BAdI Name” field.
Create an implementation with whatever naming conventions your organization uses. Eventually, you’ll be led to implementing method ENHANCE_GW_SERVICE_ENTITY_MPC in your implementation class. The simple code I used below will accomplish adding the field to the entity at runtime.
METHOD if_sra020_po_tracking_mpc~enhance_gw_service_entity_mpc. DATA: lo_property TYPE REF TO /iwbep/if_mgw_odata_property. IF iv_entity_type_name = 'POItemDetailData'. lo_property = io_entity_type->create_property( iv_property_name = 'VTWEG' iv_abap_fieldname = 'VTWEG' ). lo_property->set_nullable( abap_true ). ENDIF. ENDMETHOD.
At this point, you should be able to see a (blank) VTWEG field in your Gateway service. As part of getting the Fiori application installed (if you’re lucky to have a Ringbearer like Gavin on your team this will be done for you) you will end up with a service you can test in Gateway from t-code /IWFND/GW_CLIENT.
Getting data at runtime
METHOD if_sra020_po_tracking_dpc~change_poitemdetaildata_api. TYPES: BEGIN OF ltyp_vtweg, werks TYPE werks_d, vtweg TYPE vtwiv, END OF ltyp_vtweg. DATA: lt_vtweg TYPE TABLE OF ltyp_vtweg, ls_vtweg TYPE ltyp_vtweg, lv_vtweg TYPE vtwiv, lt_werks TYPE RANGE OF werks_d, ls_werks LIKE LINE OF lt_werks. FIELD-SYMBOLS: <ls_po_items_details> LIKE LINE OF ct_po_items_details. LOOP AT ct_po_items_details ASSIGNING <ls_po_items_details>. CLEAR ls_werks. ls_werks-sign = 'I'. ls_werks-option = 'EQ'. ls_werks-low = <ls_po_items_details>-plant. APPEND ls_werks TO lt_werks. ENDLOOP. CHECK lt_werks IS NOT INITIAL. SELECT werks vtweg INTO CORRESPONDING FIELDS OF TABLE lt_vtweg FROM t001w WHERE werks IN lt_werks. CHECK lt_vtweg IS NOT INITIAL. SORT lt_vtweg BY werks. LOOP AT ct_po_items_details ASSIGNING <ls_po_items_details>. READ TABLE lt_vtweg INTO ls_vtweg WITH KEY werks = <ls_po_items_details>-plant BINARY SEARCH. IF sy-subrc IS INITIAL. <ls_po_items_details>-vtweg = ls_vtweg-vtweg. ENDIF. ENDLOOP. ENDMETHOD.
I hope it’s obvious here that I’m just taking the PO items’ plant (weeks). And, then, using that to select on T100W to get the plant’s associated distribution channel. The changing parameter CT_PO_ITEMS_DETAILS has the fields we need to change.
After this, it’s a simple matter of rewriting the SAP kernel in Prolog, implementing your own custom RDBMS, and installing a quantum computer in your datacenter. These are, of course, optional steps – but any developer should have an easy time doing such trivial tasks.
All joking aside, after implementing the DPC BAdI you’re all done. You should see the field come into the service data and filled with the appropriate distribution channel. Proof:
Hope this helps! Remember, this doesn’t get you all the way there. You still have to update your UI5 to reveal that field on your screen. We’ll cover that soon.